I have recently learned how to 'rip' cassettes and stuff to digital so I thought I'd share some tips. I used this technique to rip cassettes but you can defintiely take this advice for ripping vinyl, the procedure is the same, it's just the set up is different. anyways, here's what you need to get started
1) you should get one of these monster icables, or something that joins the rca->1/8'' for your line-in
2) you need 'exact audio copy'
[1] to record the audio from the line-in and it helps create a 'cue sheet'
3) then cuetools
[2] to split the wav into tracks using the cue sheet
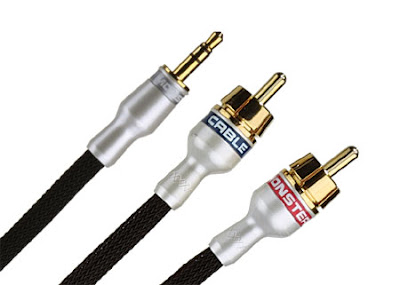
so set it up so you have the cables running into your computers mic slot or line-in and run eac -> tools -> 'record wav'. you should be able to see levels, but if you can't try going into the volume control panel and go to properties -> recording controls and select the line-in checkbox

after you get the levels, just let it record the whole tape, both sides, and then select 'process wav'
in the 'process wav' tool, first delete the leading and ending silence, but not the silence on the tape though, just the silence that is recorded when the tape wasn't even playing. then you place the 'track start' and 'gap start' markers to create a 'cue sheet'. track changes are marked by 'silence' on the tape, and the silence is much easier to see if you use the spectral view instead of the waveform view (view -> spectral).

inserting track start markers generates track n index 1 and gap markers generate track n index 0. insert a track start at the beginning of the tape's first song, and change the first marker marked track 1 index 1 into track 1 index 0 (effectively making the beginning of the recording a pregap until the first song). I also make the end of the tape silence a whole track that I edit into the cue sheet as a postgap.
go through the whole tape and then you basically get a cue sheet that looks like this

tell eac to save this cue sheet and the open it in a text editor to make sure it's correct (sometimes it doesn't export the gaps correctly, or some of the gaps correctly! see
[3] you might have to manually edit the cue sheet, the syntax is easy enough) after you have saved the cue sheet then load cuetools and use the default settings
audio output - wav
output style - gaps left out

this will generate all the tracks
Directory of C:\Music\Kites - 2007 - Deny 1\New
01.wav
02.wav
03.wav
04.wav
05.wav
06.wav
07.wav
08.wav
09.wav
CUESheet.cue
the final step is to tag the files and compress the wav. use either musicbrainz or foo_discogs to tag the files, and either foobar2000's converter utility or eac to compress the wav's.
my favorite way to do it is to load the cue sheet into foobar, select the cue sheet and tag it with the foo_discogs plugin, this writes the track metadata into the cue sheet, and then you can give the cue sheet to eac and it outputs the compressed files with properly formatted filenames!
I hope this can help you create proper rips of your music, either with your cassettes or your vinyl